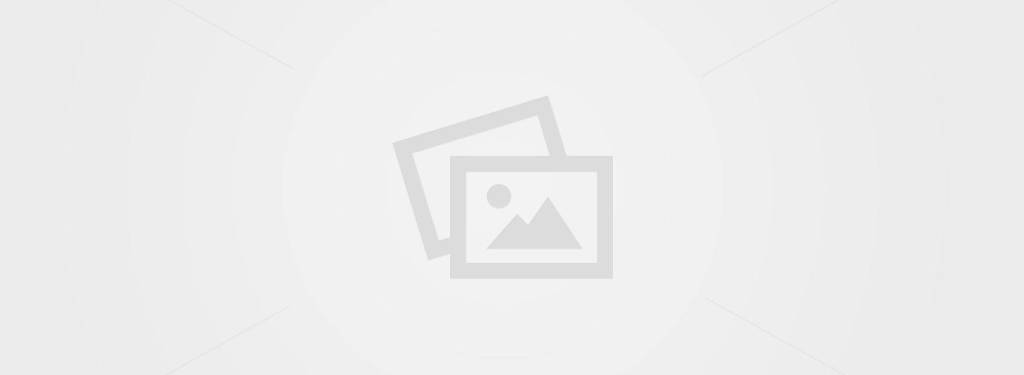
Lightning is a modern JavaScript framework designed specifically for building high-performance Smart TV apps. It offers a lightweight architecture and is optimized for low-resource environments typical of Smart TVs. Blitz is the CLI tool used for creating and managing Lightning apps. This guide will walk you through creating a Smart TV app with Lightning 3 and Blitz, including a tutorial to build a sample app.
1. Setting Up the Environment
Install Node.js
Lightning requires Node.js to run. Download and install the latest version of Node.js from nodejs.org.
Install Blitz CLI
Blitz is the command-line tool for creating and managing Lightning projects. Install it globally:
npm install -g @lightningjs/cli
Verify the installation:
lng -V
2. Create a New Lightning App
Use Blitz to create a new Lightning app:
lng create tv-sample-app
- Choose the project template (default is fine for most cases).
- Navigate to the newly created app directory:
cd tv-sample-app
3. Understand the Project Structure
After creating the app, you’ll see the following structure:
tv-sample-app/
├── src/ # Main application source files
│ ├── App.js # Entry point of the app
│ ├── components/ # Reusable UI components
│ ├── pages/ # Different screens/pages
├── static/ # Static assets like images
├── settings.json # Application settings
├── metadata.json # Metadata for the app
└── lng.config.js # Lightning configuration file
4. Running the Application
Start the development server to preview the app in a browser:
lng dev
The app will open in your default browser, showing a blank Lightning app.
5. Building a Sample App
Step 1: Modify the App Entry Point
Open src/App.js
. This is where your app’s logic begins. Replace the default content with:
import { Lightning, Router } from "@lightningjs/sdk";
export default class App extends Lightning.Component {
static _template() {
return {
Background: {
rect: true,
w: 1920,
h: 1080,
color: 0xff1a1a1a, // Dark background
},
Title: {
x: 960,
y: 100,
mount: 0.5,
text: {
text: "My Smart TV App",
fontSize: 64,
textColor: 0xffffffff,
},
},
Menu: {
x: 50,
y: 200,
children: [
{ type: MenuItem, y: 0, label: "Home" },
{ type: MenuItem, y: 80, label: "Movies" },
{ type: MenuItem, y: 160, label: "Settings" },
],
},
};
}
_init() {
this.index = 0;
this._setState("Menu");
}
static _states() {
return [
class Menu extends this {
_handleUp() {
this.index = Math.max(0, this.index - 1);
this._updateFocus();
}
_handleDown() {
this.index = Math.min(this.tag("Menu").children.length - 1, this.index + 1);
this._updateFocus();
}
_handleEnter() {
console.log("Selected:", this.tag("Menu").children[this.index].label);
}
_updateFocus() {
this.tag("Menu").children.forEach((item, idx) => {
item.patch({ focus: idx === this.index });
});
}
},
];
}
}
class MenuItem extends Lightning.Component {
static _template() {
return {
Label: {
text: { fontSize: 36, textColor: 0xff888888 },
},
};
}
set label(value) {
this._label = value;
this.tag("Label").text = value;
}
get label() {
return this._label;
}
_focus() {
this.tag("Label").setSmooth("textColor", 0xffffffff);
}
_unfocus() {
this.tag("Label").setSmooth("textColor", 0xff888888);
}
}
This creates a basic app with a title and a menu that you can navigate using the up/down buttons on the remote.
Step 2: Adding a Video Player
To add a video player, create a new component in src/components/VideoPlayer.js
:
import { Lightning } from "@lightningjs/sdk";
export default class VideoPlayer extends Lightning.Component {
static _template() {
return {
Video: {
rect: true,
w: 1280,
h: 720,
x: 320,
y: 180,
text: {
text: "Video player coming soon!",
fontSize: 36,
textColor: 0xffffffff,
},
},
};
}
}
Update the menu to navigate to the video player:
{ type: MenuItem, y: 240, label: "Player" },
Step 3: Router Integration
Enable routing in src/App.js
:
import { Router } from "@lightningjs/sdk";
Router.boot({
routes: [
{ path: "home", component: Home },
{ path: "player", component: VideoPlayer },
],
});
6. Testing Your App
Run the app again:
lng dev
Navigate through the menu and test the functionality.
7. Building and Deploying
Build the App
To generate a production build:
lng build
This creates a dist
folder with all necessary files for deployment.
Package for Platforms
Follow specific guidelines for your target Smart TV platform:
- Samsung Tizen: Use Tizen Studio to package the app.
- LG webOS: Use the webOS IDE.
- Android TV: Wrap the app into an APK with Android Studio.
8. Tips for Success
- Optimize for Performance: Avoid large assets and unnecessary re-renders.
- Test on Real Devices: Emulators are helpful but not 100% accurate.
- Follow Design Guidelines: Smart TVs require simple, bold UI designs.