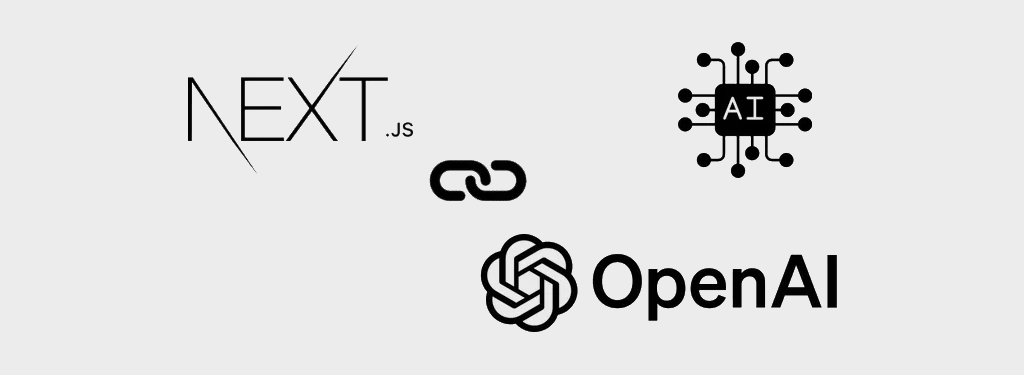
Next.js is a powerful React-based framework for building modern web applications. Combined with OpenAI’s APIs, you can supercharge your app with AI-driven capabilities like natural language processing, content generation, and more.
In this blog, we’ll walk through how to integrate OpenAI into a Next.js application, from setting up the environment to making API calls and rendering AI-generated content.
Step 1: Setting Up Your Next.js Project
1.1 Create a New Next.js App
First, initialize a new Next.js project:
npx create-next-app@latest my-openai-app
cd my-openai-app
This sets up the basic project structure with everything you need to get started.
1.2 Install Dependencies
To work with OpenAI’s API, install the official OpenAI Node.js library:
npm install openai
Step 2: Get Your OpenAI API Key
- Sign up or log in to the
OpenAI Platform
. - Navigate to the API Keys section in your account dashboard.
- Generate a new secret key and copy it. Keep this key secure and never expose it in client-side code.
Step 3: Configure Environment Variables
In your project root, create a .env.local
file to store your OpenAI API key:
OPENAI_API_KEY=your-secret-key-here
Next.js automatically loads variables from .env.local
into process.env
.
Step 4: Create an API Route for OpenAI
Since you don’t want to expose your API key to the client, use Next.js API routes to handle OpenAI API calls securely.
4.1 Create the API Route
Inside the app/api
folder, create a new file called openai.ts
:
// api/openai.ts
import { NextRequest, NextResponse } from "next/server";
import OpenAI from "openai";
export async function POST(req: NextRequest) {
const key = process.env.OPENAI_API_KEY;
if (!key) {
return NextResponse.json(
{ code: 500, error: "OPENAI_API_KEY is not set in the environment variables" },
{ status: 500 }
);
}
const openai = new OpenAI({
apiKey: key, // Set your API key
});
try {
// Parse the request body
const body = await req.json();
const { prompt } = body;
if (!prompt) {
return NextResponse.json(
{ code: 400, error: "Prompt is required" },
{ status: 400 }
);
}
const response = await openai.chat.completions.create({
model: "o1-mini-2024-09-12", // as per your plan
messages: [
{ role: "system", content: "You are a helpful assistant." },
{ role: "user", content: prompt },
],
});
const reply = response.choices[0].message.content;
return NextResponse.json({ code: 200, reply }, { status: 200 });
} catch (error) {
console.error("Error with OpenAI API:", error);
return NextResponse.json(
{ code: 500, error: "Failed to generate response" },
{ status: 500 }
);
}
}
What’s Happening Here?
- Configuration: The OpenAI client is initialized with your API key.
- API Handler: The handler accepts
POST
requests with aprompt
in the body and sends it to OpenAI’sopenai.chat.completions.create
endpoint. - Error Handling: Errors are caught and returned as a 500 response.
Step 5: Build the Frontend
Next, create a simple frontend to send user inputs to the API and display AI-generated responses.
5.1 Create a Page
Edit the default app/page.tsx
to include a form and a display area:
"use client";
import { FormEvent, useState } from "react";
export default function Home() {
const [prompt, setPrompt] = useState("");
const [response, setResponse] = useState("");
const [loading, setLoading] = useState(false);
const handleSubmit = async (e: FormEvent<HTMLFormElement>) => {
e.preventDefault();
setLoading(true);
setResponse("");
try {
const res = await fetch("/api/openai", {
method: "POST",
headers: {
"Content-Type": "application/json",
},
body: JSON.stringify({ prompt }),
});
const data = await res.json();
if (res.ok) {
setResponse(data.result);
} else {
setResponse(`Error: ${data.error}`);
}
} catch (error) {
setResponse(`Error: ${error}`);
} finally {
setLoading(false);
}
};
return (
<div style={{ padding: "2rem", maxWidth: "600px", margin: "auto" }}>
<h1>Ranjeet Rajput AI Tool</h1>
<form onSubmit={handleSubmit}>
<textarea
value={prompt}
onChange={(e) => setPrompt(e.target.value)}
rows={4}
style={{ width: "100%", padding: "0.5rem" }}
placeholder="Ask something..."
/>
<button type="submit" style={{ marginTop: "1rem" }} disabled={loading}>
{loading ? "Loading..." : "Submit"}
</button>
</form>
{response && (
<div style={{ marginTop: "2rem", padding: "1rem", border: "1px solid #ccc" }}>
<h3>Response:</h3>
<p>{response}</p>
</div>
)}
</div>
);
}
What’s Happening Here?
- The form collects user input (
prompt
) and sends it to the/api/openai
route usingfetch
. - The AI’s response is displayed below the form.
Step 6: Test Your Application
- Run your development server:
npm run dev
- Open your browser and navigate to http://localhost:3000.
- Enter a question or command in the text area and submit it. Watch as OpenAI generates a response.
Optional Enhancements
1. Use a Custom Model
If you’ve fine-tuned a model in OpenAI, replace "text-davinci-003"
with your model’s name in the API route.
2. Add Loading Indicators
Enhance the user experience by adding loading spinners or animations while waiting for the API response.
3. Rate Limiting
Protect your API from abuse by adding rate-limiting middleware, such as next-rate-limit
.
4. Deploy Your App
Deploy your Next.js app to platforms like Vercel or Netlify. Ensure that your environment variables (API key) are configured securely in the hosting platform.
Conclusion
Integrating OpenAI into a Next.js application is straightforward and opens up endless possibilities. With just a few steps, you can harness the power of AI to build smarter, more interactive applications.
Ready to start? Try building your own AI-powered app today, and let us know what you create! If you have any questions or need help : LINKED IN POST