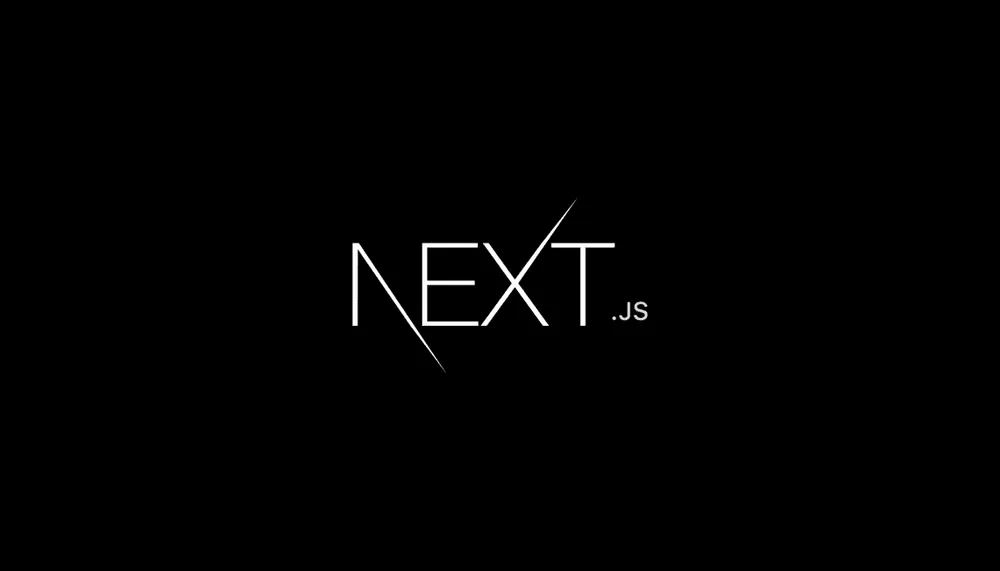
Introduction
Setting up a Next.js project isnโt just about installing dependencies; itโs about creating a maintainable, high-quality foundation. In this guide, weโll cover a proven setup for essential tools like Prettier, ESLint, Husky, and Commitlint to ensure consistency, catch issues early, and improve team workflows, helping you stay organized and productive.
Project Setup
Node & PNPM Versions
For this guide, Iโm using Node.js 20.18.0
and PNPM 9.12.3
.
Start by creating a new Next.js project:
pnpm create next-app@latest
Name your project when prompted and select “Yes” for the ESLint setup:
โ What is your project named? โฆ next-template
โ Would you like to use TypeScript? โฆ No / Yes
โ Would you like to use ESLint? โฆ No / Yes
โ Would you like to use Tailwind CSS? โฆ No / Yes
โ Would you like your code inside a `src/` directory? โฆ No / Yes
โ Would you like to use App Router? (recommended) โฆ No / Yes
โ Would you like to use Turbopack for next dev? โฆ No / Yes
โ Would you like to customize the import alias (@/* by default)? โฆ No / Yes
After the prompts, create-next-app
generates a folder named after your project and installs the required dependencies.
Tip: Use the --yes
option to skip prompts and apply default values:
pnpm create next-app@latest --yes next-template
Engine Locking
Ensuring consistent Node.js versions across environments is crucial. Specify the supported Node.js version in your package.json
:
{
"engines": {
"node": ">=18.8.x"
}
}
Additionally, add a .npmrc
file:
engine-strict=true
This configuration ensures that incompatible Node.js versions trigger an error during installation.
Commit your changes:
git commit -m 'build: added engine locking'
ESLint
ESLint comes pre-configured with Next.js. You can make it stricter by updating .eslintrc.json
:
{
"extends": ["next/core-web-vitals"],
"rules": {
"no-console": ["error", { "allow": ["info", "warn", "error"] }]
}
}
For Tailwind CSS linting:
pnpm add -D eslint-plugin-tailwindcss
Update your .eslintrc.json
:
{
"extends": [
"next/core-web-vitals",
"plugin:tailwindcss/recommended"
],
"rules": {
"tailwindcss/classnames-order": "error"
}
}
Commit your changes:
git commit -m 'build: added eslint rules and Tailwind plugin'
Prettier
Install Prettier:
pnpm add --save-dev --save-exact prettier
Add a .prettierrc
configuration:
{
"semi": false,
"singleQuote": false,
"tabWidth": 2
}
Ignore unnecessary files using .prettierignore
:
node_modules
.next
Add Prettier scripts to package.json
:
{
"scripts": {
"format": "prettier --write .",
"format:check": "prettier --check ."
}
}
For Tailwind CSS class sorting:
pnpm add -D prettier-plugin-tailwindcss
Update .prettierrc
:
{
"plugins": ["prettier-plugin-tailwindcss"]
}
Commit your changes:
git commit -m 'build: added Prettier setup with Tailwind plugin'
Git Hooks with Husky
Install Husky:
pnpm add --save-dev husky
pnpm exec husky init
Add a pre-commit hook:
echo "pnpm lint-staged" > .husky/pre-commit
Install lint-staged
for staged file linting:
pnpm add -D lint-staged
Configure .lintstagedrc.js
:
const buildEslintCommand = (filenames) =>
`next lint --fix --file ${filenames.join(' --file ')}`;
module.exports = {
"*.{js,jsx,ts,tsx}": [buildEslintCommand],
"*.{json,css,md}": ["prettier --write"]
};
Commit your changes:
git commit -m 'build: added Husky and lint-staged setup'
VS Code Setup
Install recommended extensions:
- Prettier
- ESLint
- Tailwind CSS IntelliSense
Add workspace settings to .vscode/settings.json
:
{
"editor.defaultFormatter": "esbenp.prettier-vscode",
"editor.formatOnSave": true
}
Commit your changes:
git commit -m 'build: added VS Code workspace settings'
Recap
A solid setup accelerates development and ensures consistency in team environments. By following these practices, youโll save time, avoid errors, and create a maintainable codebase.
Thatโs it for now. Happy coding! โ๏ธ