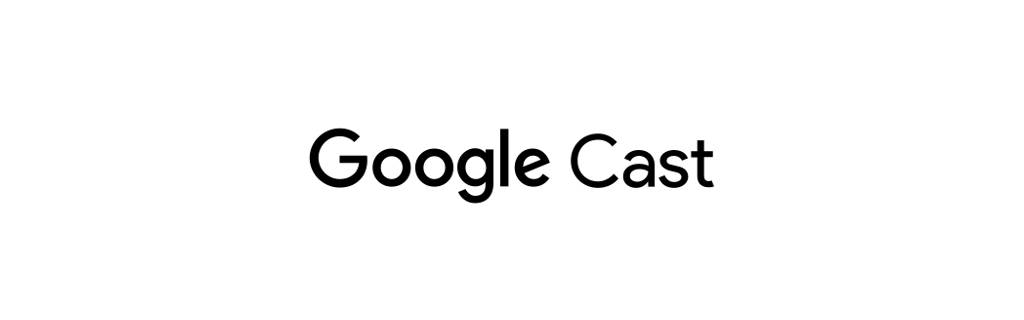
As streaming content becomes a cornerstone of modern entertainment, the demand for seamless and efficient media delivery has skyrocketed. Google Castโs Web Receiver API allows developers to create robust receiver applications tailored to stream media effortlessly to Chromecast and other Cast-enabled devices. This guide walks you through the steps to build a Cast Web Receiver API application from scratch.
Prerequisites
Before diving into development, ensure you have the following:
- Basic Knowledge:
- JavaScript, HTML, and CSS.
- Familiarity with RESTful APIs.
- Tools:
- A code editor (e.g., Visual Studio Code).
- Google Chrome for debugging.
- A Chromecast device or Cast-enabled device for testing.
- Google Cast SDK:
- Sign up for a Google Cast Developer account.
- Register your Cast application in the Google Cast SDK Developer Console.
Step 1: Setting Up the Project
1.1 Create a Directory Structure
Organize your project with the following structure:
project-root/
|-- index.html
|-- css/
| |-- receiver.css
|-- js/
| |-- receiver.js
1.2 Initialize the Project
Set up a basic HTML file:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Cast Receiver</title>
<link rel="stylesheet" href="css/receiver.css">
<script src="//www.gstatic.com/cast/sdk/libs/caf_receiver/v3/cast_receiver_framework.js"></script>
</head>
<body>
<cast-media-player></cast-media-player>
<script src="js/receiver.js" type="module"></script>
</body>
</html>
Step 2: Implement the Receiver Logic
2.1 Initialize CastReceiverContext
In receiver.js
, initialize the Cast Receiver context:
'use strict';
const context = cast.framework.CastReceiverContext.getInstance();
const playerManager = context.getPlayerManager();
context.start();
2.2 Customize Player Behavior
Add event listeners for player lifecycle events to customize behavior:
playerManager.setMessageInterceptor(cast.framework.messages.MessageType.LOAD, (loadRequest) => {
console.log('Load request received:', loadRequest);
// Validate or modify the media request here
return loadRequest;
});
2.3 Implement License Handling (if DRM is required)
For DRM-protected content, configure license handling:
const playbackConfig = new cast.framework.PlaybackConfig();
playbackConfig.licenseRequestHandler = (requestInfo) => {
requestInfo.headers = {
...requestInfo.headers,
Authorization: 'Bearer YOUR_ACCESS_TOKEN'
};
};
context.setPlaybackConfig(playbackConfig);
Step 3: Style the Receiver
3.1 Add Custom CSS
Define a custom layout and appearance for the receiver:
/* receiver.css */
body {
margin: 0;
background-color: #000;
color: #fff;
font-family: Arial, sans-serif;
display: flex;
justify-content: center;
align-items: center;
height: 100vh;
}
cast-media-player {
width: 100%;
height: 100%;
}
Step 4: Test Your Receiver
4.1 Launch the Receiver
- Host your application on a web server (e.g., use
http-server
or deploy to Firebase). - Register the hosted URL in the Google Cast SDK Developer Console.
4.2 Debug with Chrome
- Open
chrome://inspect
in Google Chrome. - Connect to your Cast device and inspect the running receiver app.
Step 5: Deployment
5.1 Finalize and Deploy
- Ensure your app adheres to Googleโs receiver guidelines.
- Deploy to a production-ready web server.
5.2 Register the App
- Submit your app for approval in the Google Cast Developer Console.
Conclusion
By following this guide, youโve successfully created a basic Cast Web Receiver API application. While this serves as a solid foundation, consider adding advanced features like analytics tracking, custom UI components, and enhanced playback capabilities to make your app truly stand out.
Happy Casting!