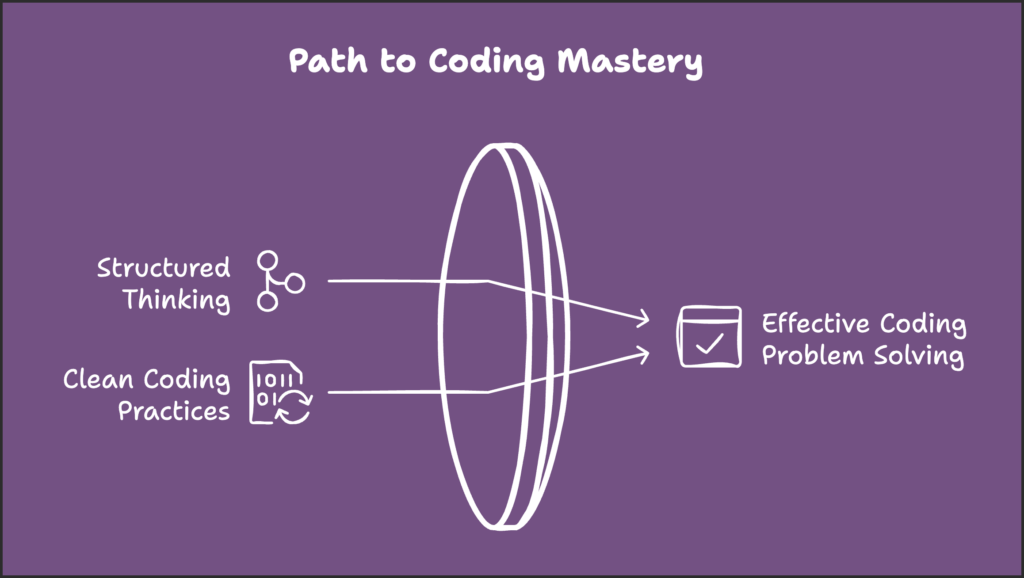
Coding problems are a staple of programming, from job interviews to real-world application development. Solving them effectively requires structured thinking and clean coding practices. Hereโs a streamlined approach to tackle coding challenges with an emphasis on clean JavaScript examples.
1. Understand the Problem
Before you dive into coding, ensure you fully understand the problem.
Steps:
- Read the problem statement multiple times.
- Identify the inputs, outputs, and constraints.
- Break the problem into smaller, digestible pieces.
- Clarify any ambiguities or assumptions.
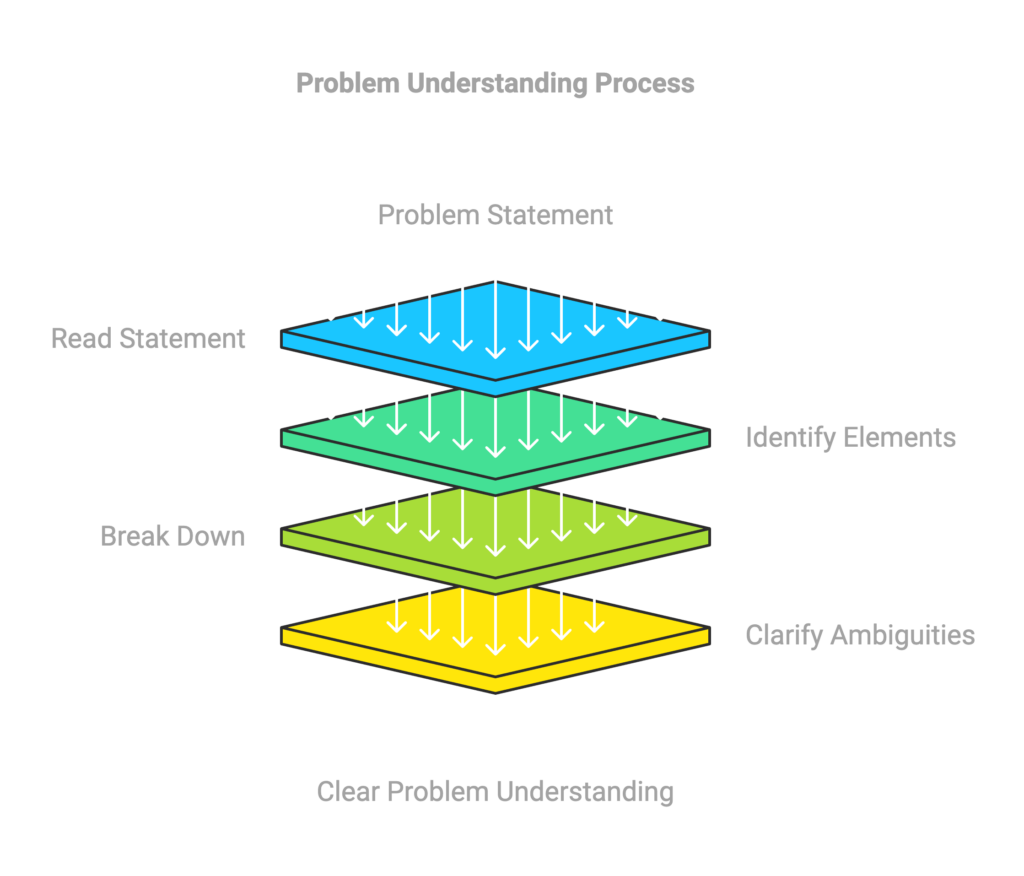
Example: If tasked to โreverse a string,โ ensure you understand if the input can include special characters or if itโs case-sensitive.
2. Plan Your Approach
Think before you code. A solid plan saves time and prevents errors.
Steps:
- Brainstorm possible solutions (e.g., brute force, recursion, or optimized algorithms).
- Choose a method that aligns with the problemโs constraints.
- Draft pseudocode to map out your logic.
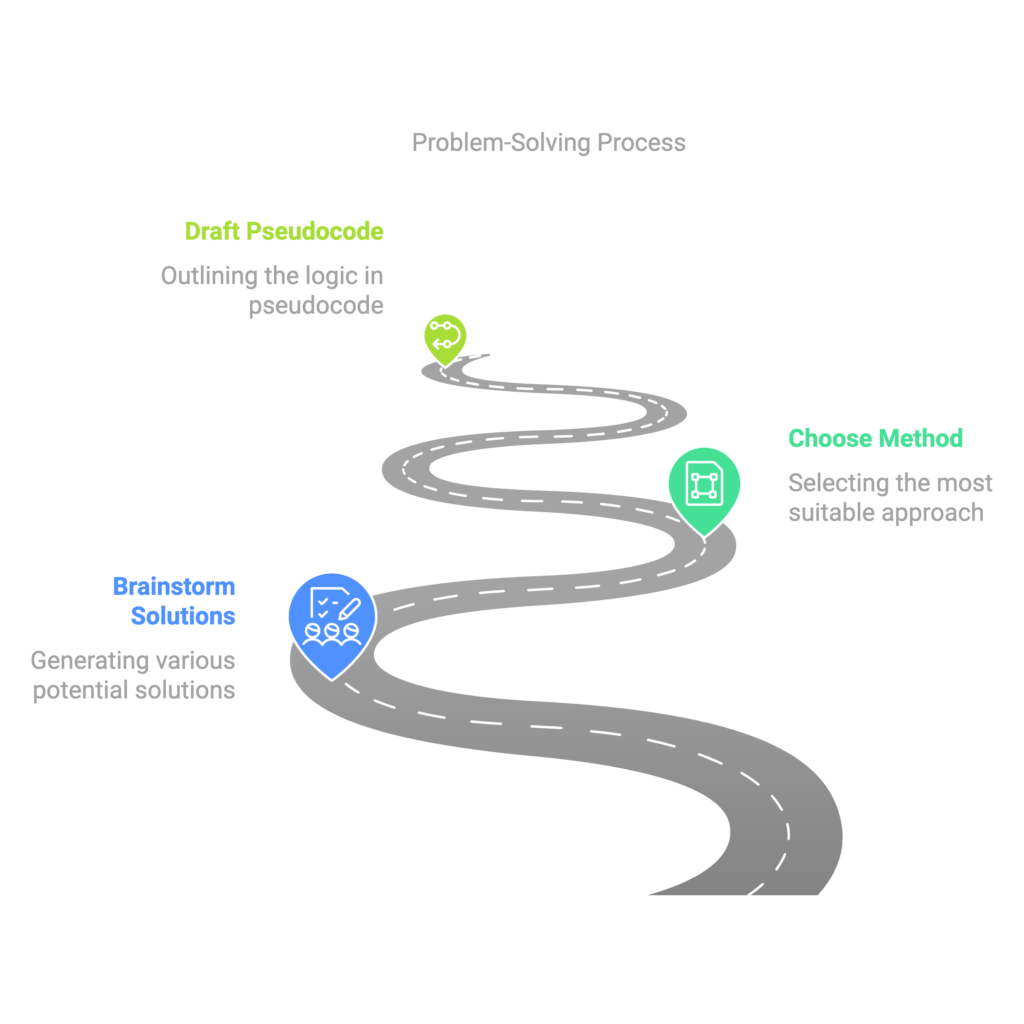
Pro Tip: Focus on edge cases early, such as empty inputs or maximum constraints.
3. Break Down the Problem
Simplify complex problems by dividing them into smaller parts.
Steps:
- Identify sub-problems or intermediate steps.
- Solve each sub-problem independently.
- Combine the results to build your final solution.
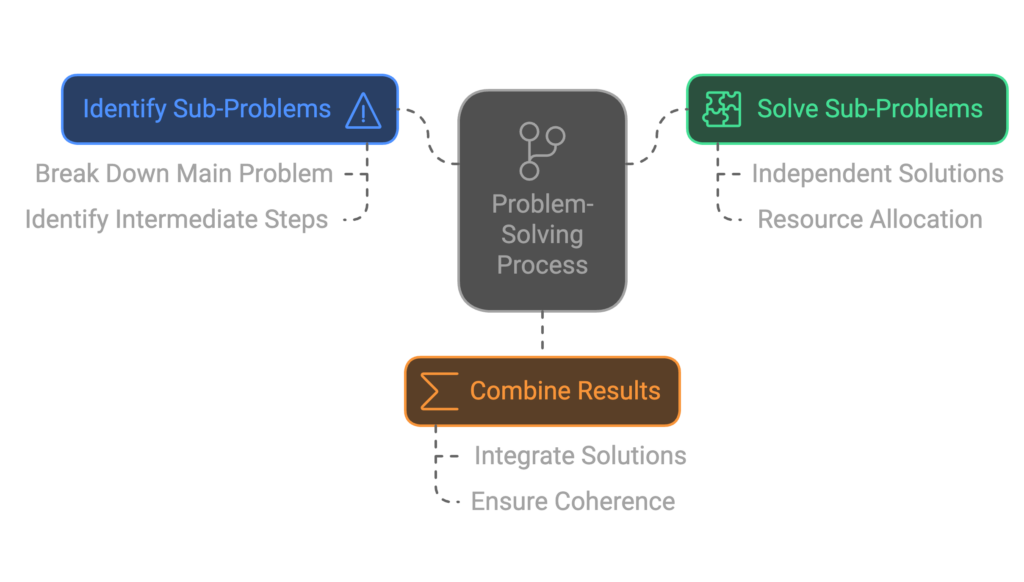
Example in JS:
javascriptCopy code// Problem: Check if a number is a palindrome
function isPalindrome(num) {
const str = num.toString();
return str === str.split('').reverse().join('');
}
// Break it into steps:
// 1. Convert the number to a string.
// 2. Reverse the string.
// 3. Compare the original and reversed strings.
4. Write Clean Code
Clean code is readable, modular, and easy to maintain. Focus on simplicity and organization.
Tips for Writing Clean Code:
- Use descriptive names for variables and functions.
- Keep functions small and focused on a single task.
- Avoid unnecessary comments by writing self-explanatory code.
Example:
javascriptCopy code// Clean Code Example: Finding the Maximum Number in an Array
function findMax(arr) {
if (!Array.isArray(arr) || arr.length === 0) {
throw new Error('Input must be a non-empty array');
}
return Math.max(...arr);
}
// Usage
const numbers = [3, 5, 7, 2, 8];
console.log(findMax(numbers)); // Output: 8
Key Practices:
- Validate inputs.
- Handle edge cases (e.g., empty arrays).
- Keep functions concise.
5. Test Your Code
Testing is essential to ensure reliability.
Steps:
- Test with given examples.
- Create edge cases to verify robustness.
- Test performance with large inputs.
Example Test Cases for findMax
:
javascriptCopy codeconsole.log(findMax([1, 2, 3])); // Output: 3
console.log(findMax([-1, -2, -3])); // Output: -1
console.log(findMax([100])); // Output: 100
6. Optimize Your Solution
Once your code works, analyze its efficiency and look for improvements.
Metrics to Consider:
- Time Complexity: How does runtime scale with input size?
- Space Complexity: How much memory does your solution use?
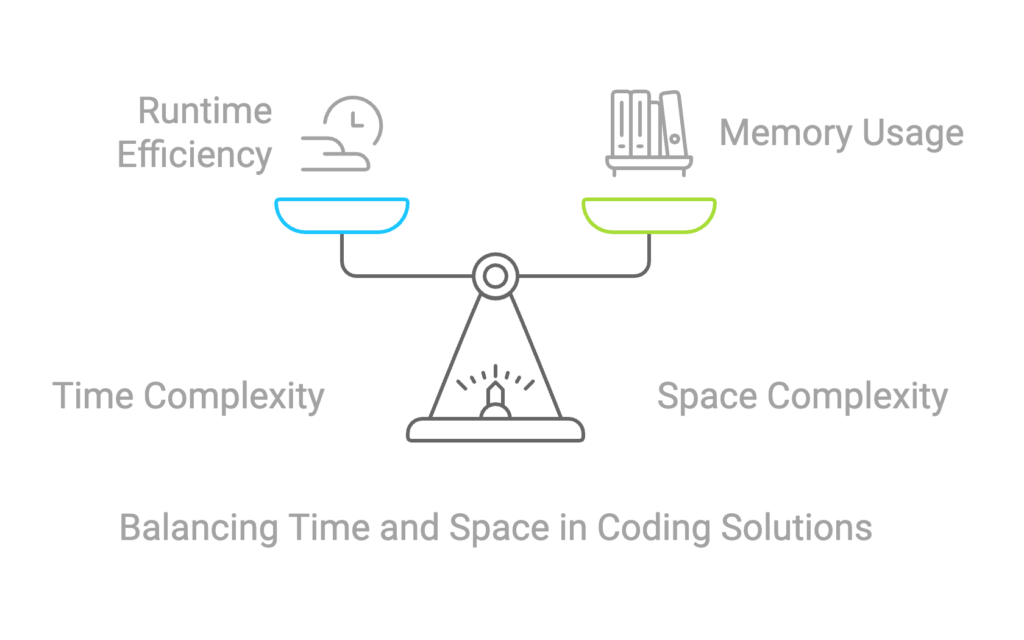
Example Optimization: A brute force solution to find duplicate elements in an array may have O(n2)O(n^2)O(n2) complexity. Using a Set
, you can reduce it to O(n)O(n)O(n).
javascriptCopy codefunction findDuplicates(arr) {
const seen = new Set();
const duplicates = new Set();
for (const num of arr) {
if (seen.has(num)) {
duplicates.add(num);
} else {
seen.add(num);
}
}
return [...duplicates];
}
7. Learn from Mistakes
Every problem you solve is an opportunity to learn. Reflect on:
- Where you struggled.
- How others approached the same problem.
- How you can apply these lessons in future challenges.
8. Practice Regularly
Consistency is key to mastering coding challenges. Platforms like LeetCode, HackerRank, and Codewars provide excellent practice opportunities.
Pro Tip: Practice a variety of problem types, including arrays, strings, recursion, and dynamic programming.
Final Thoughts
Solving coding problems is as much about mindset as it is about technical skill. A systematic approach, combined with clean, efficient code, sets you up for success. Embrace the learning process, and with practice, youโll find yourself solving problems faster and more effectively.
Happy Coding!